GeoParse
It's all about points
lines
and polygons 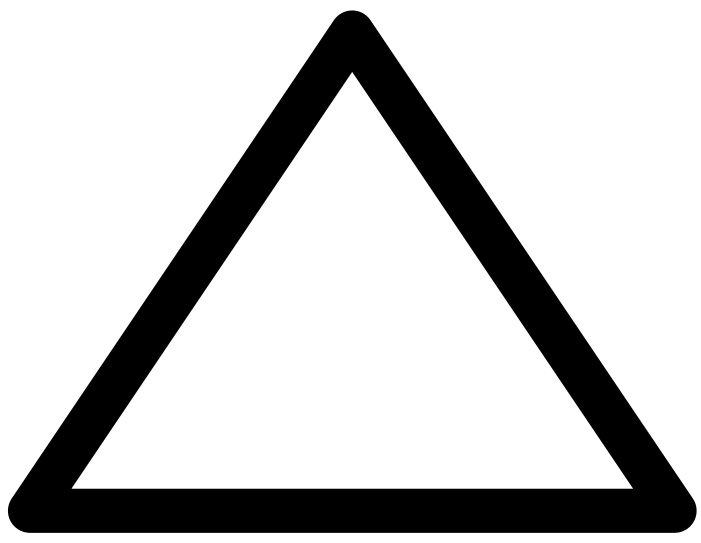
Website¶
Visualization¶
This notebook demonstrates how to display:
- Points, lines, and polygons.
- Their heatmaps and clusters.
- Their coverage areas using geospatial cells such as H3, S2, and Geohash.
- Geospatial cells on a map based on their indexes.
- OSM roads and buildings using their IDs.
In [1]:
import sys
import os
sys.path.append(os.path.abspath("../geoparse/"))
In [2]:
import warnings
import geopandas as gpd
import pandas as pd
from geoparse import plp
warnings.filterwarnings("ignore")
Read from CSV file¶
In [3]:
df = pd.read_csv("data/great_britain_road_casualties-2023.csv")
df.head()
Out[3]:
date | time | lat | lon | number_of_vehicles | number_of_casualties | speed_limit | |
---|---|---|---|---|---|---|---|
0 | 03/01/2023 | 19:12 | 51.356551 | -0.097759 | 1 | 1 | 30 |
1 | 07/01/2023 | 10:05 | 51.593701 | 0.022379 | 1 | 1 | 30 |
2 | 14/01/2023 | 16:15 | 51.466689 | -0.011289 | 1 | 1 | 20 |
3 | 15/01/2023 | 19:51 | 51.671577 | -0.037543 | 1 | 1 | 30 |
4 | 16/01/2023 | 19:22 | 51.447944 | 0.117279 | 2 | 1 | 30 |
In [4]:
len(df)
Out[4]:
1522
In [5]:
plp(df)
Out[5]:
Make this Notebook Trusted to load map: File -> Trust Notebook
In [6]:
plp(df, heatmap=True, cluster=True)
Out[6]:
Make this Notebook Trusted to load map: File -> Trust Notebook
In [7]:
plp(df, point_color="speed_limit", point_popup={"Speed limit": "speed_limit"})
Out[7]:
Make this Notebook Trusted to load map: File -> Trust Notebook
In [ ]:
Read a Polygon from Geo File¶
In [8]:
gdf = gpd.read_file("data/london.geojson")
gdf
Out[8]:
name | geometry | |
---|---|---|
0 | London | POLYGON ((-0.51035 51.46822, -0.51028 51.46837... |
In [9]:
plp(gdf)
Out[9]:
Make this Notebook Trusted to load map: File -> Trust Notebook
In [10]:
plp(gdf, centroid=True)
Out[10]:
Make this Notebook Trusted to load map: File -> Trust Notebook
In [11]:
plp(gdf, h3_res=6)
Out[11]:
Make this Notebook Trusted to load map: File -> Trust Notebook
In [12]:
plp(gdf, h3_res=10, compact=True)
Out[12]:
Make this Notebook Trusted to load map: File -> Trust Notebook
In [13]:
plp(gdf, s2_res=12)
Out[13]:
Make this Notebook Trusted to load map: File -> Trust Notebook
In [14]:
plp(gdf, s2_res=14, compact=True)
Out[14]:
Make this Notebook Trusted to load map: File -> Trust Notebook
In [15]:
plp(gdf, geohash_res=5)
Out[15]:
Make this Notebook Trusted to load map: File -> Trust Notebook
In [16]:
plp(gdf, geohash_res=7, compact=True)
Out[16]:
Make this Notebook Trusted to load map: File -> Trust Notebook